🚀DevOps Zero to Hero: 💡Day 19 — Test Automation🚦
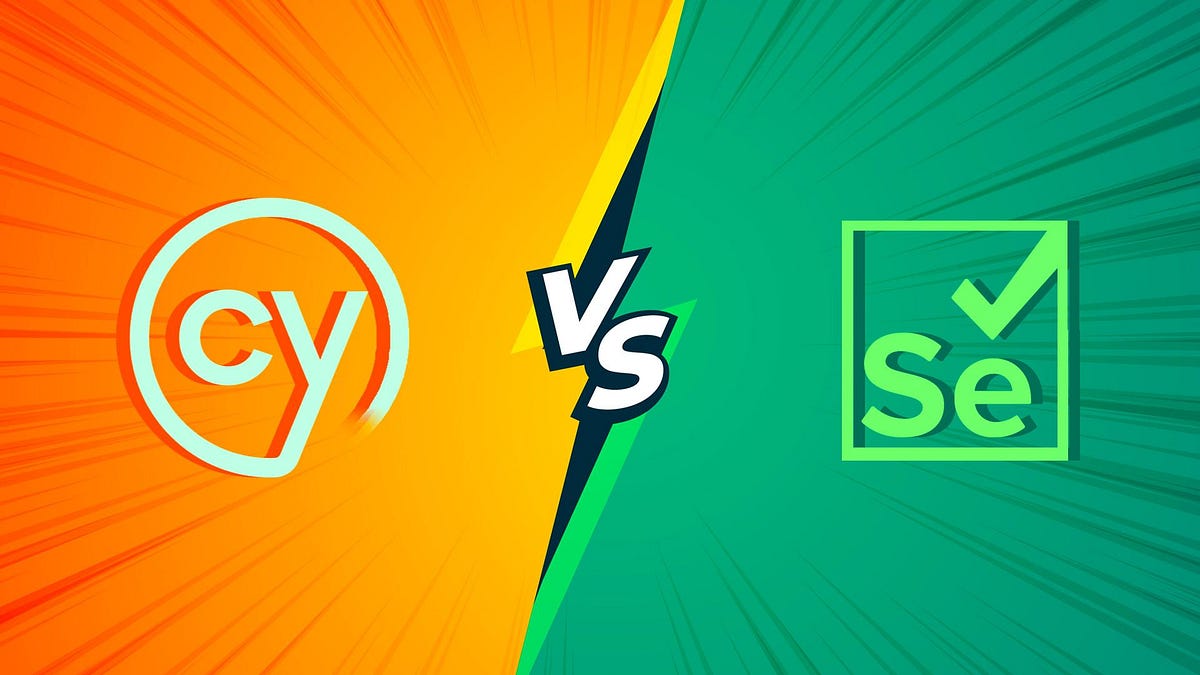
Welcome to Day 19 of our 30-day course dedicated to mastering Test Automation! Today, we will delve into the realm of test automation frameworks, such as Selenium and Cypress. Our focus will be on designing and implementing automated tests for web applications and seamlessly integrating them into your CI/CD pipeline.
Introduction to Test Automation Frameworks:
In the realm of software testing, test automation frameworks are instrumental in facilitating efficient and reliable automated testing. These frameworks offer guidelines, best practices, and tools to structure and execute automated tests effectively. They simplify the complexities of test automation, making it user-friendly for testers and developers to create and maintain automated test suites.
1. Selenium:
Selenium stands out as a widely used open-source test automation framework for web applications. It empowers testers to automate interactions with web browsers and conduct seamless functional testing. Supporting multiple programming languages like Java, Python, C#, JavaScript, Ruby, and more, Selenium caters to testers and developers with diverse language preferences.
Key Features of Selenium:
- Browser Automation: Control web browsers programmatically to simulate user interactions.
- Cross-Browser Testing: Supports various browsers, including Chrome, Firefox, Safari, Edge, and Internet Explorer.
- Element Locators: Provides diverse locators for identifying elements on web pages.
- Parallel Execution: Executes tests in parallel, reducing overall test execution time.
- Integration with Testing Frameworks: Integrates seamlessly with testing frameworks like TestNG and JUnit.
2. Cypress:
Cypress emerges as a modern and developer-friendly end-to-end testing framework designed primarily for web applications. Written in JavaScript, Cypress boasts a straightforward API, making it easy for developers to write and maintain tests. Unlike traditional testing tools, Cypress operates directly in the browser, facilitating close interaction with the application under test.
Key Features of Cypress:
- Real-Time Reloading: Provides real-time reloading as tests are written, enhancing the development and debugging process.
- Time Travel: Allows pausing and debugging tests at any point during execution.
- Automatic Waiting: Waits for elements to appear on the page before interaction, eliminating the need for explicit waits.
- Debuggability: Offers comprehensive debugging tools like Chrome DevTools, logging detailed information about test runs.
- Snapshot and Video Recording: Captures screenshots and records videos of test runs, aiding in diagnosing failures.
Choosing Between Selenium and Cypress:
The choice between Selenium and Cypress depends on project requirements and team expertise. Consider factors such as application type, programming language preferences, testing speed, debugging capabilities, and community support.
Here are some factors to consider:
Application Type: If you primarily work with traditional web applications and require cross-browser testing, Selenium might be a more suitable choice. On the other hand, if you’re building modern web applications and prefer a more developer-friendly experience, Cypress might be a better fit.
Programming Language: If you have a strong background in a specific programming language, consider whether Selenium’s language support aligns with your expertise.
Testing Speed: Cypress offers fast test execution due to its architecture, while Selenium might take longer for complex test suites.
Debugging: Cypress provides advanced debugging capabilities, making it easier to identify and troubleshoot issues.
Community and Support: Selenium has been around for a longer time and has a larger community and more extensive documentation. However, Cypress has gained significant popularity and community support as well.
Ultimately, both Selenium and Cypress are powerful test automation frameworks, and the choice depends on your specific project needs and team preferences.
Designing and Implementing Automated Tests for Web Applications:
Let’s walk through an example project for designing and implementing automated tests for a simple web application using Selenium (with Python) and Cypress (with JavaScript).
Example Project: Automated Tests for a ToDo List Web Application.
For this example, we’ll create automated tests for a basic ToDo list web application. The application allows users to add tasks, mark them as completed, and delete tasks.
Prerequisites:
a. Install Python
b. Install Selenium WebDriver for Python
c. Download the appropriate WebDriver (e.g., ChromeDriver) and add it to your system’s PATH
Test Scenario: Verify that tasks can be added, marked as completed, and deleted in the ToDo list application.
1. Create a new Python file named test_todo_list_selenium.py.
2. Implement the test cases using Selenium:
from selenium import webdriver
import time
# Initialize the WebDriver (using Chrome in this example)
driver = webdriver.Chrome()
# Open the ToDo list application
driver.get("https://exampletodolistapp.com")
# Test Case 1: Add a task
task_input = driver.find_element_by_id("new-task")
add_button = driver.find_element_by_id("add-button")
task_input.send_keys("Buy groceries")
add_button.click()
# Verify that the task has been added to the list
task_list = driver.find_element_by_id("task-list")
assert "Buy groceries" in task_list.text
# Test Case 2: Mark task as completed
complete_checkbox = driver.find_element_by_xpath("//span[text()='Buy groceries']/preceding-sibling::input[@type='checkbox']")
complete_checkbox.click()
# Verify that the task is marked as completed
assert "completed" in complete_checkbox.get_attribute("class")
# Test Case 3: Delete the task
delete_button = driver.find_element_by_xpath("//span[text()='Buy groceries']/following-sibling::button")
delete_button.click()
# Verify that the task has been removed from the list
assert "Buy groceries" not in task_list.text
# Close the browser
driver.quit()
3. Run the test using python test_todo_list_selenium.py.
Designing and Implementing Automated Tests with Cypress (JavaScript)
Prerequisites:
a. Install Node.js
b. Install Cypress
Test Scenario: Verify that tasks can be added, marked as completed, and deleted in the ToDo list application.
1. Create a new folder for the Cypress project and navigate into it.
2. Initialize a new Cypress project using the following command:
npx cypress open
3. After the Cypress application launches, you’ll find the cypress/integration folder.
4. Create a new file named todo_list_cypress.spec.js.
5. Implement the test cases using Cypress:
describe('ToDo List Tests', () => {
beforeEach(() => {
cy.visit('https://exampletodolistapp.com');
});
it('Adds a task', () => {
cy.get('#new-task').type('Buy groceries');
cy.get('#add-button').click();
cy.contains('Buy groceries').should('be.visible');
});
it('Marks a task as completed', () => {
cy.get('#new-task').type('Buy groceries');
cy.get('#add-button').click();
cy.get('input[type="checkbox"]').check();
cy.get('input[type="checkbox"]').should('be.checked');
});
it('Deletes a task', () => {
cy.get('#new-task').type('Buy groceries');
cy.get('#add-button').click();
cy.get('button').click();
cy.contains('Buy groceries').should('not.exist');
});
});
6. Click on the test file (todo_list_cypress.spec.js) in the Cypress application to run the test.
Cypress will open a browser window, and you will see the automated tests executing. You can also view detailed logs, screenshots, and videos of the test execution in the Cypress application.
In this example project, we demonstrated how to design and implement automated tests for a ToDo list web application using both Selenium with Python and Cypress with JavaScript. Selenium offers flexibility across various programming languages and browsers, while Cypress provides a more streamlined and developer-friendly experience for modern web applications.
Integrating Automated Tests into the CI/CD Pipeline:
Integrating automated tests into the CI/CD pipeline is a pivotal step in the software development process. It ensures that tests are executed automatically upon code changes, facilitating early issue detection and resolution.
Let’s elaborate on the steps to integrate automated tests into the CI/CD pipeline:
1. Set Up a Version Control System:
The first step is to set up a version control system (VCS) like Git. Version control allows you to manage changes to your codebase, collaborate with team members, and keep track of different versions of your software.
2. Create a CI/CD Pipeline:
Next, you need to set up a CI/CD pipeline using a CI/CD tool of your choice. Popular CI/CD tools include Jenkins, GitLab CI/CD, Travis CI, CircleCI, and GitHub Actions.
The CI/CD pipeline consists of a series of automated steps that are triggered whenever changes are pushed to the version control repository. The pipeline typically includes steps like building the application, running automated tests, deploying the application to staging or production environments, and generating reports.
3. Configuring the CI/CD Pipeline for Automated Tests:
To integrate automated tests into the CI/CD pipeline, you need to configure the pipeline to execute the test suite automatically after each code commit or pull request.
Here are the general steps for this configuration:
Install Dependencies: Ensure that the required dependencies (e.g., programming languages, testing frameworks, and drivers for Selenium) are installed on the CI/CD server or agent.
Check Out Code: The CI/CD pipeline should check out the latest code from the version control repository.
Build the Application: If necessary, build the application to create an executable or distributable artifact.
Run Automated Tests: Execute the automated test suite using the appropriate testing framework. For example, if you’re using Selenium with Python, run the Python script that contains your Selenium tests.
Reporting and Exit Status: Capture the test results and generate test reports. Most testing frameworks provide options to output test results in a machine-readable format (e.g., JUnit XML). Additionally, ensure that the pipeline exits with an appropriate exit status based on the test results (e.g., exit with code 0 for success and a non-zero code for test failures).
4. Handling Test Results:
The CI/CD pipeline should handle the test results appropriately. If any tests fail, developers should be notified immediately so they can address the issues. Some CI/CD tools provide built-in integrations with messaging platforms like Slack or email services to send notifications.
5. Parallel and Distributed Testing (Optional):
For larger projects with a significant number of automated tests, consider running tests in parallel or distributing them across multiple agents or machines to speed up test execution.
6. Post-Build Actions:
Depending on your workflow, you might also consider triggering deployments to staging or production environments after a successful build and test run. However, it’s essential to ensure that your automated tests provide adequate coverage and validation before proceeding with deployment.
Integrating automated tests into the CI/CD pipeline is a powerful practice that can significantly improve the quality and reliability of your software. It helps catch bugs early, provides rapid feedback to developers, and ensures that your application remains in a deployable state at all times.
By configuring your CI/CD pipeline to run automated tests automatically, you enable a seamless integration of testing into your development workflow, making it easier to deliver high-quality software to end-users with greater confidence.
Conclusion:
Mastering test automation with frameworks like Selenium and Cypress is paramount for modern software development. By seamlessly integrating automated tests into your CI/CD pipeline, you elevate your development workflow, ensuring the reliability of your web applications.
Congratulations on completing Day 19 of our course! Tomorrow, we will explore deployment strategies. Happy Learning!